6.00.1x - Week 3
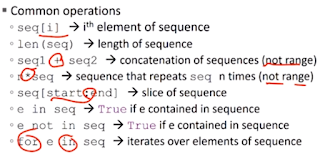
3.5.1 Tuples ordered sequence of mixed element types immutable, represented with parentheses () must add comma ',' at the end of 1 element tuple, convenient for swapping variable values 3.5.2 Lists ordered sequence of mixed element types mutable, represented with square brackets [] https://docs.python.org/3/tutorial/datastructures.html 3.5.3 List Operations append, extend (change the list) del, pop, remove, list (convert string to list) sort vs. sorted, reverse range returns something that behaves like a tuple or a list 3.5.4 Mutation, Aliasing, Cloning clone: chill = old[:] sort: mutates the list, returns nothing sorted: does not mutate list, returns a new sorted list 3.5.5 Functions as Objects Can pass function name as parameter map(abs, [1, -2, -3, -4] => [1, 2, 3, 4] map(min, L1, L2) 3.6.1 Quick Review 3.6.2 Dictionaries instead of using multiple lists, use dictionary represented with curly braces: {} mutable, keys, values, no order key: