6.00.1x - Week 5
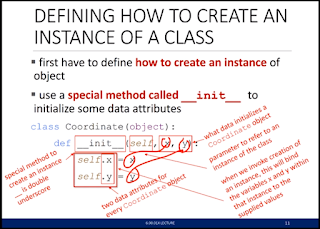
5.9.1 Object Oriented Programming Object: 3 pieces: type, internal data representation, and set of procedures for interaction with the object Instance is a particular type of object. 1234 is an instace of an int, a = 'hello' is an instance of a string Data abstraction: represent internal data (private) using attributes, interface for interacting with object through methods Advantages of OOP: bundle data into packages, divide-and-conquer development, and ability to reuse code 5.9.2 Class Instances class Coordinate(object): class: class definition class name: Coordinate class parent: object 5.9.3 Methods def __init__(self, attr): special method (constructor) to create an instance and initialize data (optional) __str__: equivalent to toString() in other languages to print custom string for class representational invariant: enforce a particular rule by code 5.9.5 Why OOP? 5.9.6 Hierarchies 5.9.7 Class Variables 5.9.9 Generators \